How to configure ESLint and Prettier with VSCode
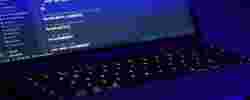

Coding is, most of the time, not as difficult as you may think. But one thing is harder than coding : strictness. Indeed, having the same coding style within an entire project is really hard.
In a production project, you will need to have this strictness. That's why many javascript developers use ESLint and Prettier and, in this article, I'm going to show you how to configure them properly.
In this example, we'll assume that you are starting a brand new javascript project. But all the explanations I'll give you will work just as well for an old project.
Install the VSCode plugins
The first thing you'll need to do in order to use Prettier and ESLint within VSCode in your project is to download two plugins :
- Eslint (by Dirk Baeumer)
- Prettier - Code formatter (by Prettier)
😄 Obviously
Install the dependencies
Then, you'll need to install ESLint and Prettier as project dependencies. More precisely dev dependencies.
To install it, just type the following command on your terminal.
npm i -D eslint prettier
The -D
flag means that these two dependencies will be installed as dev dependencies. We are actually installing them as dev dependencies because they are not needed in production. That's why we specify the -D
flag.
After that, we'll need to install two libraries to create a bridge between ESLint and Prettier.
npm i -D eslint-config-prettier eslint-plugin-prettier
The next step is to initialize and configure ESLint to make it work for your project.
Configure ESLint
Now that you have all the required dependencies, the next step is to configure ESLint.
To do that, type the following command.
./node_modules/eslint/bin/eslint.js --init
This command will ask you multiple questions in order to know how you want to configure ESLint for your project.
- The first question is about how you want to use ESLint. I personally like the last option :
To check syntax, find problems, and enforce code style
. - The next question is about the type of modules you are using. Depending on the nature of your project, you can choose one of the three options.
- Then, it will ask you if you are using a javascript framework like React or Vue.
- The next one is about Typescript.
- Then, it will ask you where does your code run ?
- The next is really important. It will ask you if you want to choose a popular coding style. For me, the one that fits the most my needs is the AirBnb coding style. So for this question I usually choose
Use a popular style guide
. - Then, I choose the AirBnb style guide.
- Finally, I save my configuration in a
Json
file. - Then, they will ask you if you want to install all the dependencies, say
yes
and you will be ready to go.
At the end, this script should create a .eslintrc.json
file with your entire configuration.
Now, the last step is to make it work together.
Configuration files
ESLint configuration file
In order to make ESLint and Prettier work together, we'll need to make some modifications in the .eslintrc.json
file. You have to add a new rule, a new plugin and an extension.
In the extends
section, you need to add prettier
like this :
"extends": [
...
"prettier"
]
Then, we'll need to add the prettier
plugin like this :
"plugins": [
...
"prettier"
]
The last thing is to indicate that you want to execute your prettier rules when ESLint detects an error on your coding style. This can be done by adding the prettier/prettier
rule like this :
"rules": {
...
"prettier/prettier": "error"
}
Now lets configure the prettier behavior.
Prettier configuration file
In order to configure prettier with your own rules, you first need to create a .prettierrc
file.
Then, you can add the content you want to fit your needs. For me, the configuration I personally like is the following.
{
"semi": true,
"singleQuote": false,
"tabWidth": 3,
"endOfLine": "lf",
"printWidth": 999999
}
Now, when ESLint detects an error in your coding style, it will automatically fix it using the prettier rules you've set above.
The last step is to fix every coding style issues when you save the file you're working on.
VSCode configuration file
As well as the prettier configuration file, you'll nee to create it. It needs to be located in .vscode/settings.json
.
Then you can put the following content to allow VSCode to fix all the issues using ESLint and Prettier.
{
"[javascript]": {
"editor.formatOnSave": false
},
"editor.codeActionsOnSave": {
"source.fixAll": true
}
}
These few lines are the minimum to make it work. Then you can add some more lines to fit your needs like so.
{
"editor.tabSize": 3,
"[javascript]": {
"editor.formatOnSave": false
},
"editor.codeActionsOnSave": {
"source.fixAll": true
},
"files.eol": "\n",
"prettier.printWidth": 999999
}
Overview
Now, if you create a javascript file with some coding style issues like the code below :
var hello = 'World !'
And then you save by pressing Ctrl+s
, ESLint and Prettier should be executed to fix every issue. You should come up with the following code after saving it.
const hello = "World !";
As you can see, it has automatically changed every single quote to double quote, it added a semicolon at the end of each line and it converted the var
into a const
.
You want more details about the AirBnb coding guide, you should take a look at the github repository : https://github.com/airbnb/javascript#readme
Conclusion
Now that everything is configure and up and running, your coding style should be the same in every files. At least if you use javascript. The process can be applied for a typescript project, React or vue.
I hope it helped you and good luck on your coding journey👍
Cover by Mohammad Rahmani